Explore 20+ Django projects with source code to enhance your skills. Perfect for beginners and advanced developers looking to learn by example.

Are you looking to level up your Django skills? Whether you're a beginner or an experienced developer, working on real projects is one of the best ways to learn. In this post, we’ll showcase 20+ Django projects complete with source code. These projects will help you understand how to build and manage web applications using Django, a powerful Python framework.
Table of Contents
Prerequisites
Before you start working on these Django projects, make sure you have the following prerequisites:
- Python: Django is built on Python, so you'll need Python installed on your system. You can download it from the official Python website.
- Pip: Pip is the package installer for Python. It is usually included with Python, but you can install it separately if needed.
- Virtual Environment: It’s a good practice to use a virtual environment to manage dependencies. This helps keep your project isolated from other Python projects on your system.
- Django: Install Django using pip once your virtual environment is set up.
- Code Editor: Use a code editor like Visual Studio Code, PyCharm, or any other that you are comfortable with.
Setting Up the Development Environment
Follow these steps to set up your development environment:
- Install Python: If you haven’t installed Python yet, download it from the official Python website. Follow the installation instructions for your operating system.
- Create a Virtual Environment:
- Open your terminal or command prompt.
- Navigate to the directory where you want to store your projects.
- Run the following command to create a virtual environment:
- Replace myenv with the name you want for your virtual environment.
- Activate the Virtual Environment:
- On Windows:
- On macOS/Linux:
- Install Django:
- With your virtual environment activated, install Django by running:
- Start a New Django Project:
- To create a new Django project, run:
- Replace myproject with your desired project name.
python -m venv myenv
myenv\Scripts\activate
source myenv/bin/activate
pip install django
django-admin startproject myproject
1. Calculator
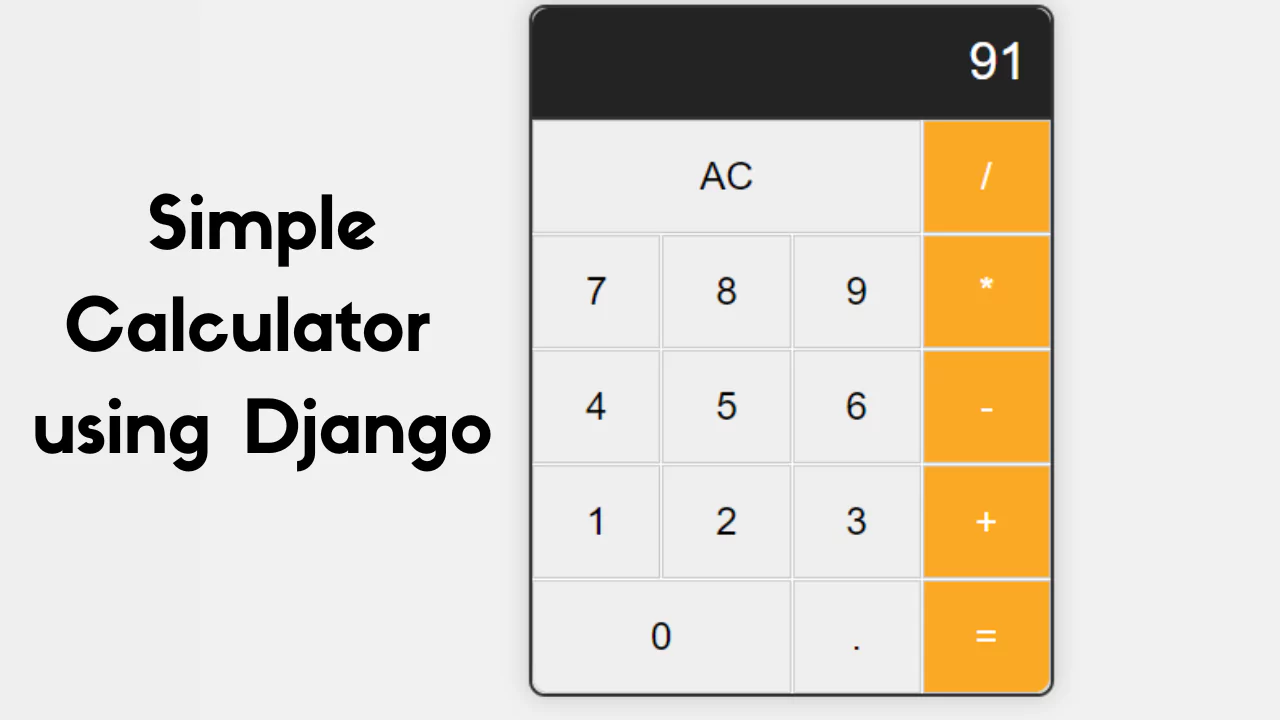
In this project, you'll build a basic calculator using Django. It's a great way to learn how to handle user input and perform calculations on the server side. This project will help you understand Django forms and how to display dynamic results.
2. Age Calculator
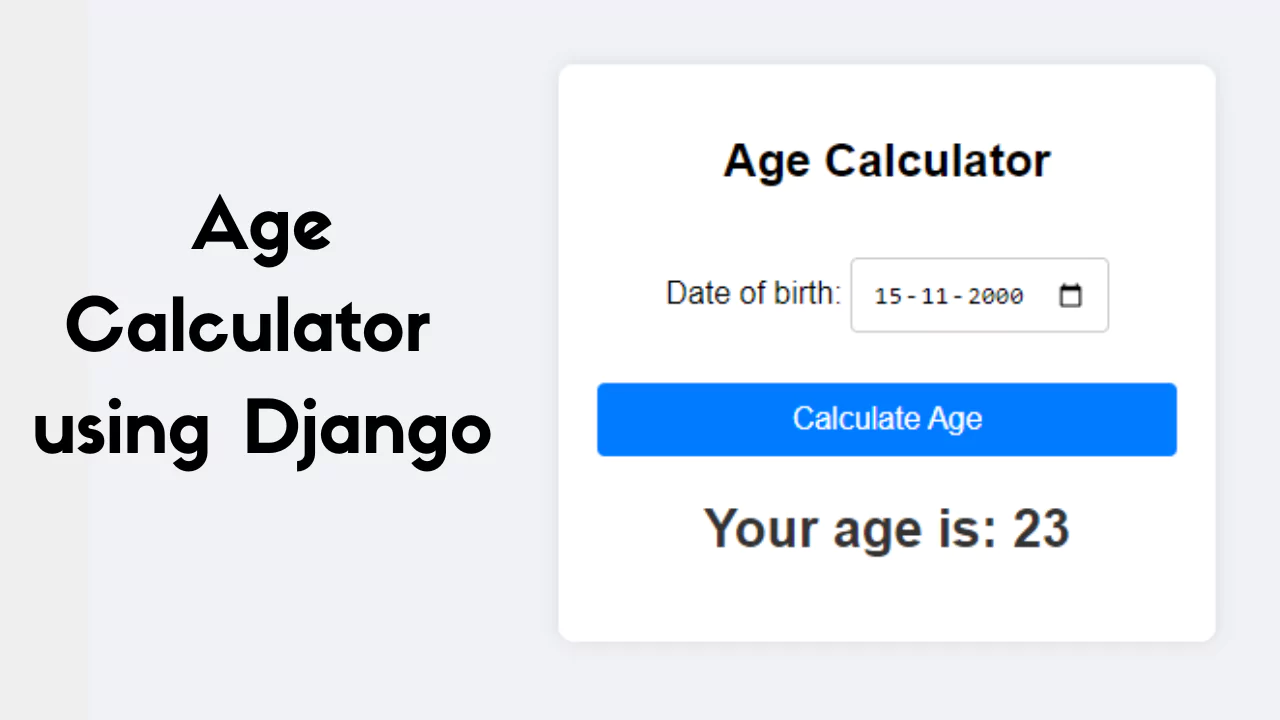
Create an age calculator with Django that calculates a person's age based on their birth date. This project will teach you how to work with date and time in Django and display the results to the user.
3. BMI Calculator
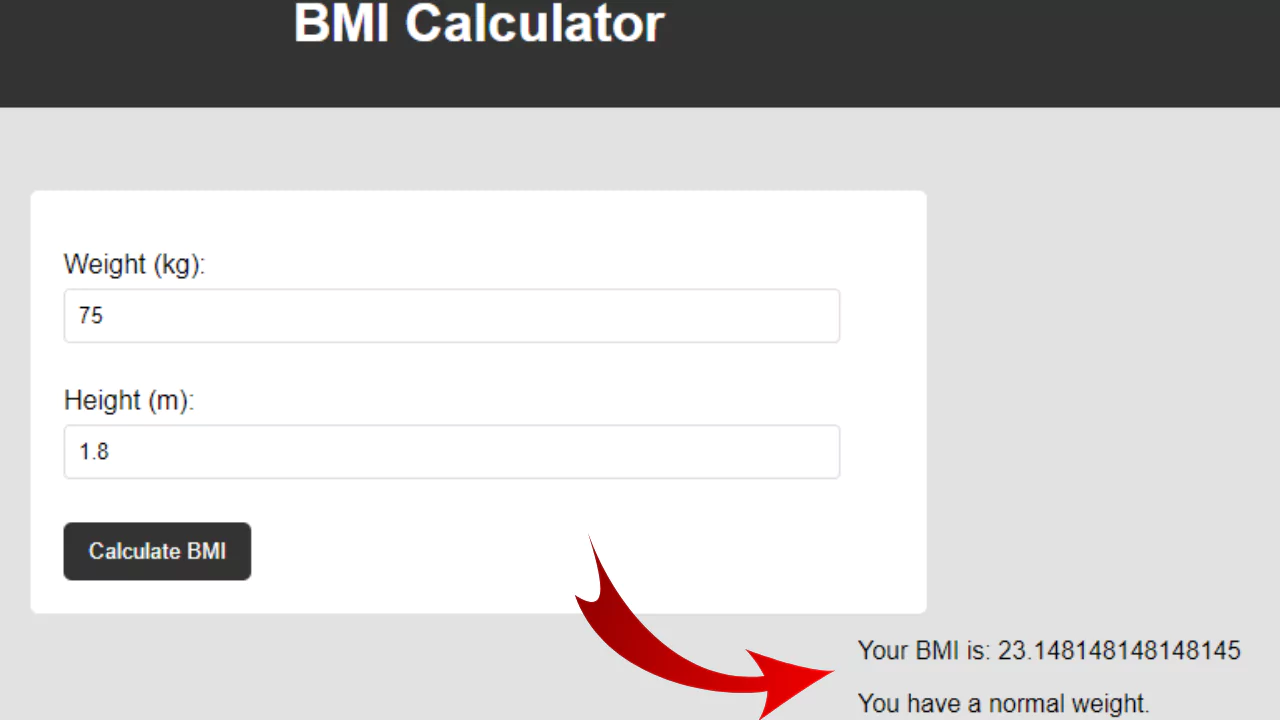
Build a BMI calculator using Django that takes user input for height and weight and calculates their Body Mass Index. This project will guide you through handling user input, performing calculations, and displaying the results in a user-friendly manner.
4. Password Generator
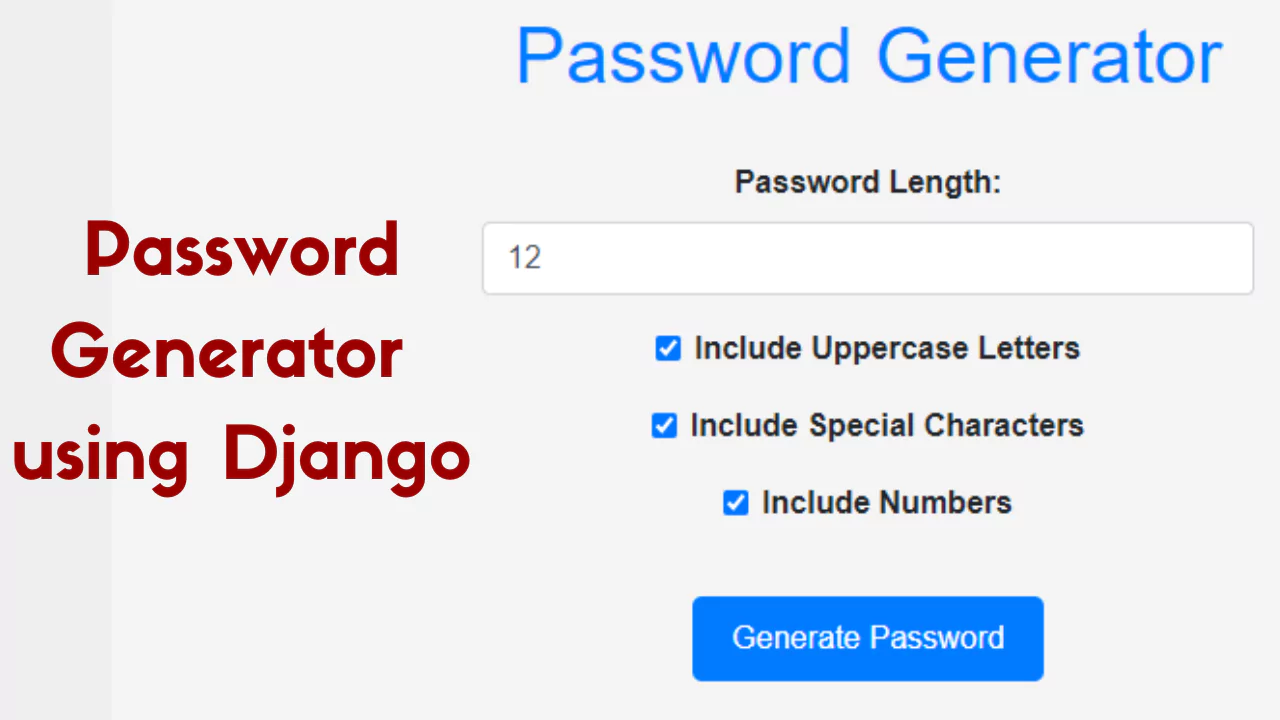
Develop a Django-based password generator that creates secure, random passwords. This project will introduce you to generating and handling dynamic content in Django, as well as ensuring security best practices.
5. Password Generator and Strength Checker
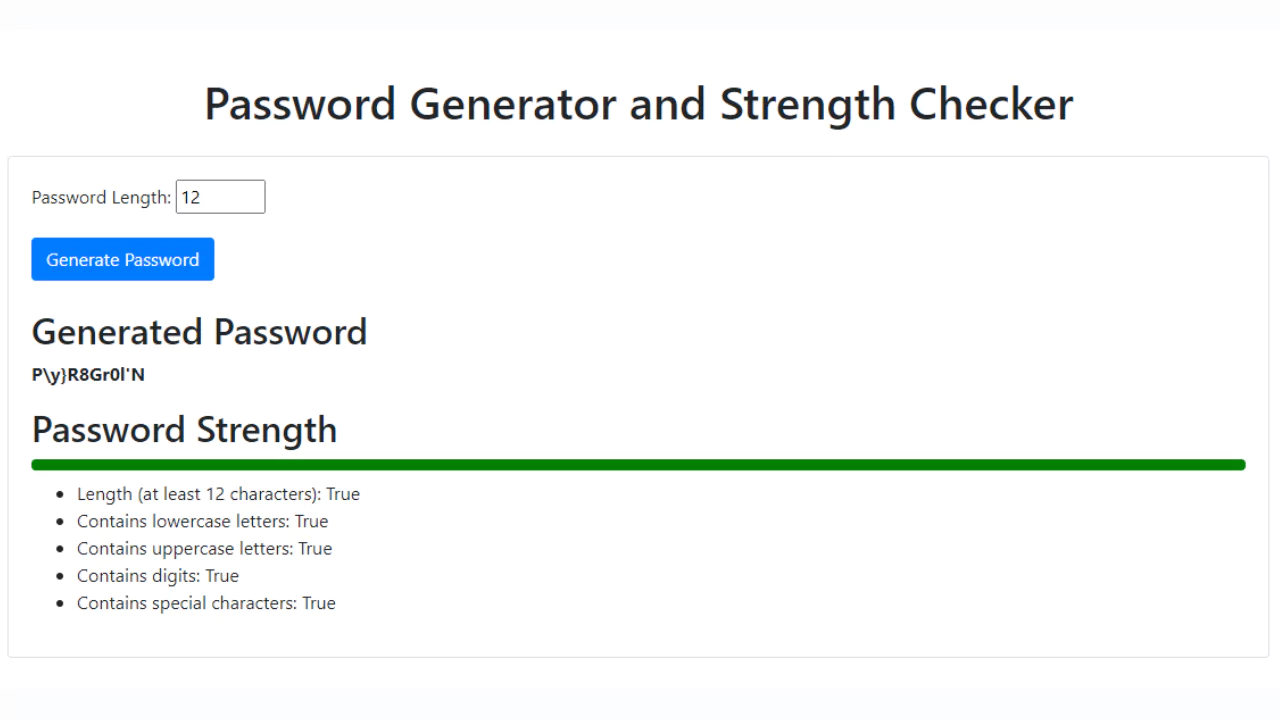
Enhance your Django password generator by adding a feature that checks and displays the strength of the generated passwords. This project will deepen your understanding of client-server interactions and user experience considerations.
6. QR Code Generator
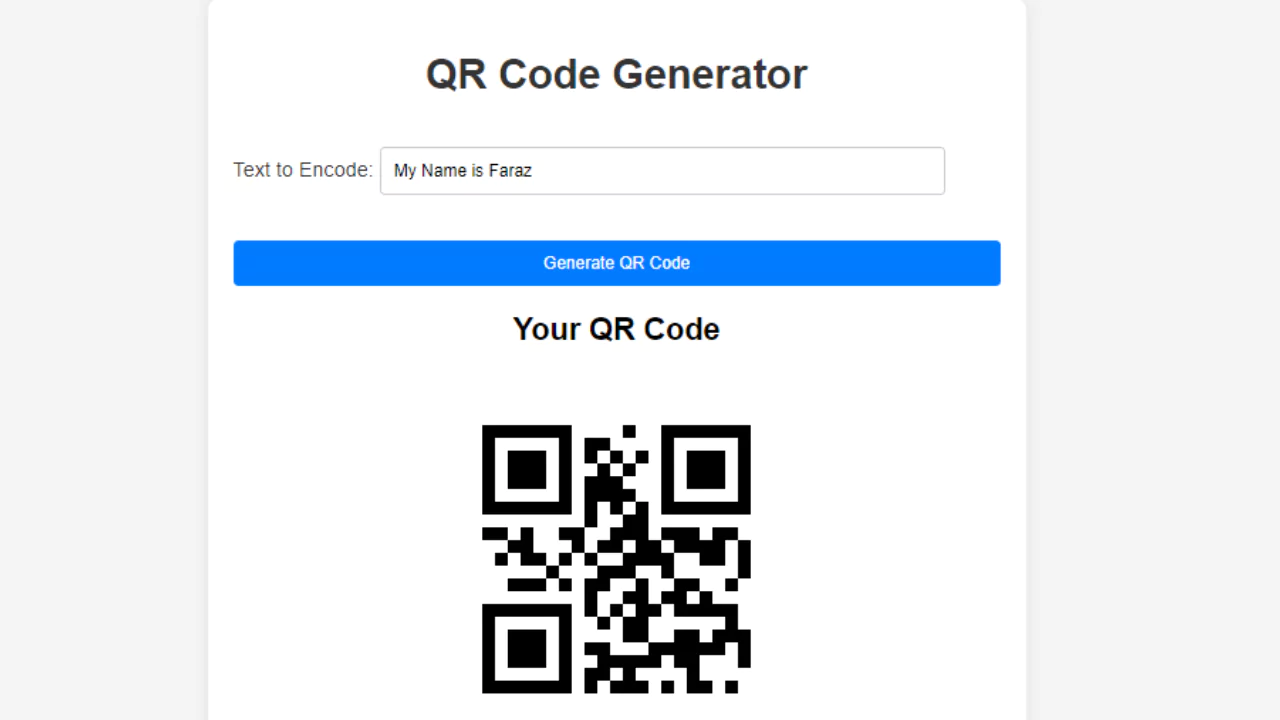
In this project, you'll create a Django web app that generates QR codes from user-provided data. This is an excellent way to learn about integrating third-party libraries and providing instant feedback to users.
7. Joke Generator
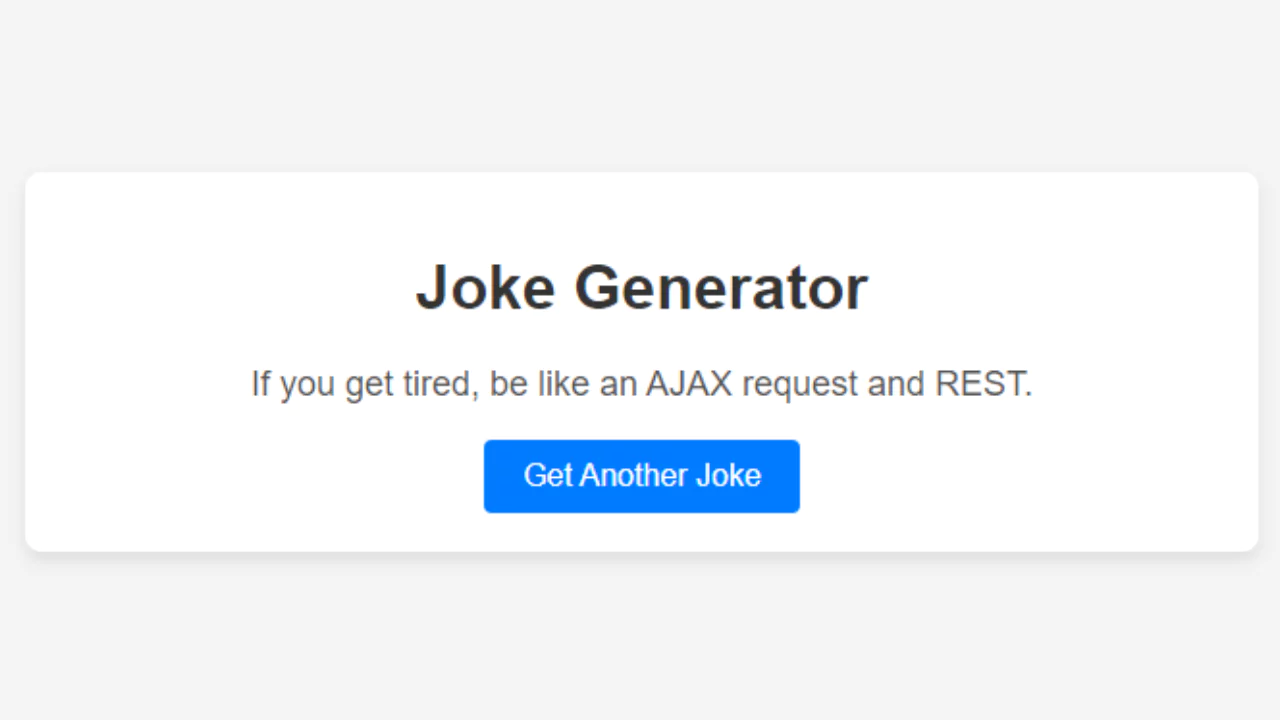
Build a joke generator in Django that fetches and displays random jokes from an API. This project will help you understand how to make API requests in Django and display the results dynamically to users.
8. Word Counter
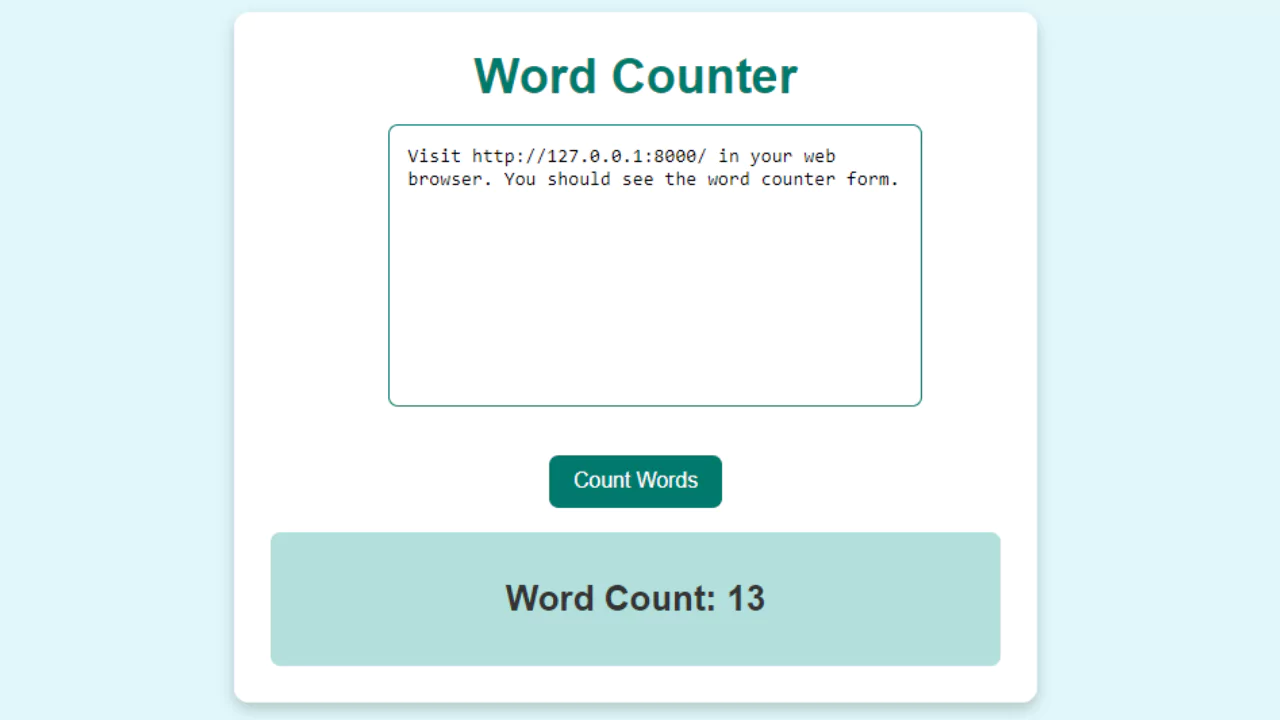
Create a word counter tool using Django that allows users to input text and get detailed statistics, such as word and character counts. This project is perfect for learning about text processing and user interaction in Django.
9. Countdown Timer
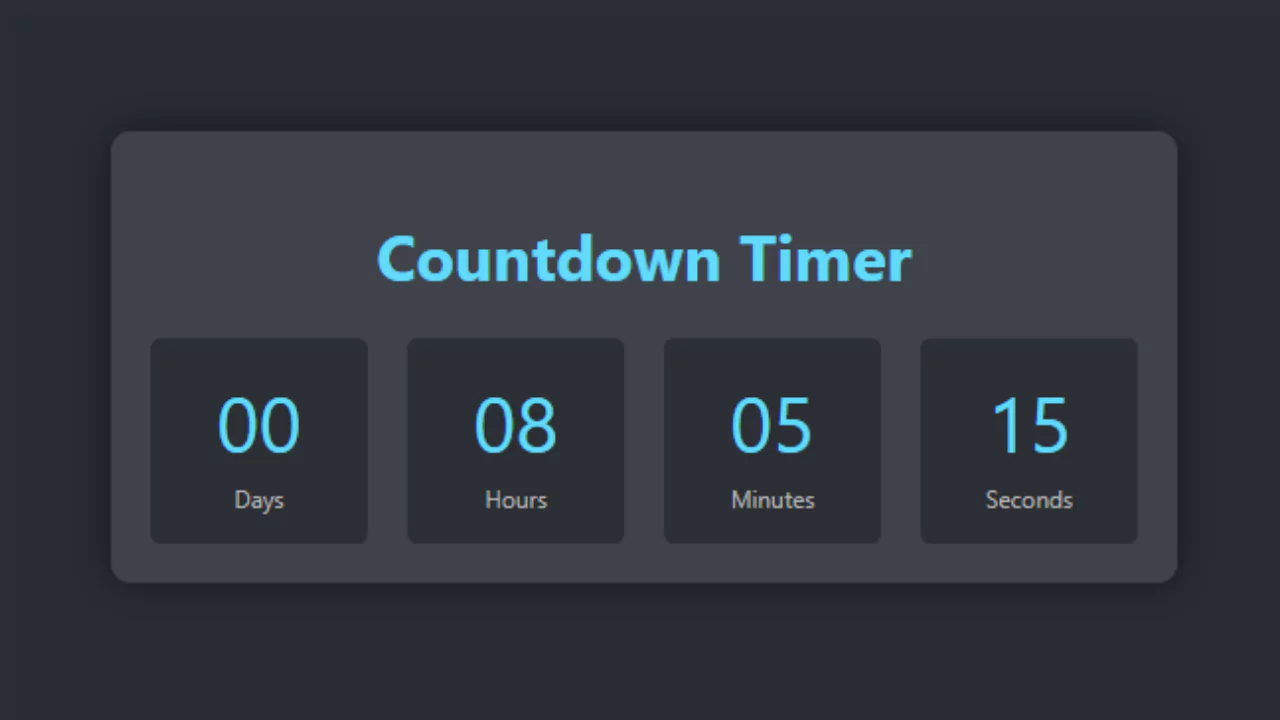
Develop a countdown timer with Django that counts down to a specific event or time. This project will teach you about managing time-related data and creating dynamic, real-time features in your Django applications.
10. Quiz App
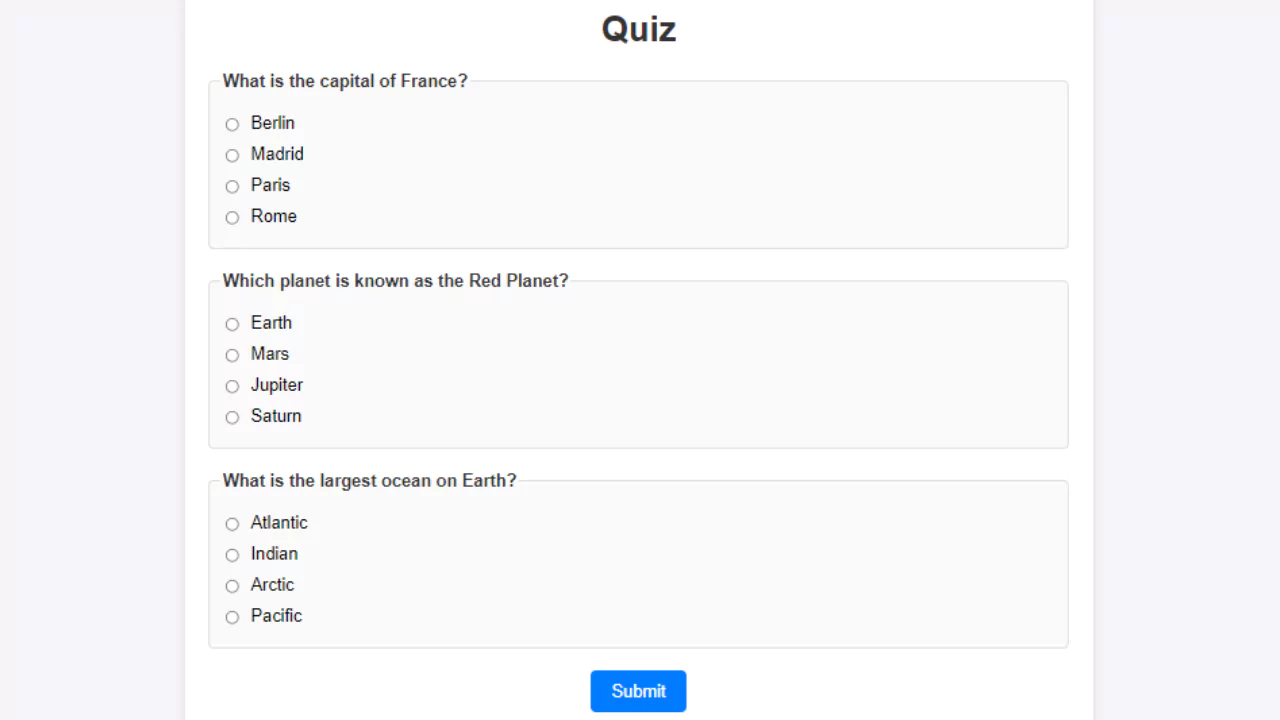
Build a quiz application using Django where users can answer questions and get their scores instantly. This project will help you learn about handling user sessions, managing data, and creating interactive content.
11. Translator Web App
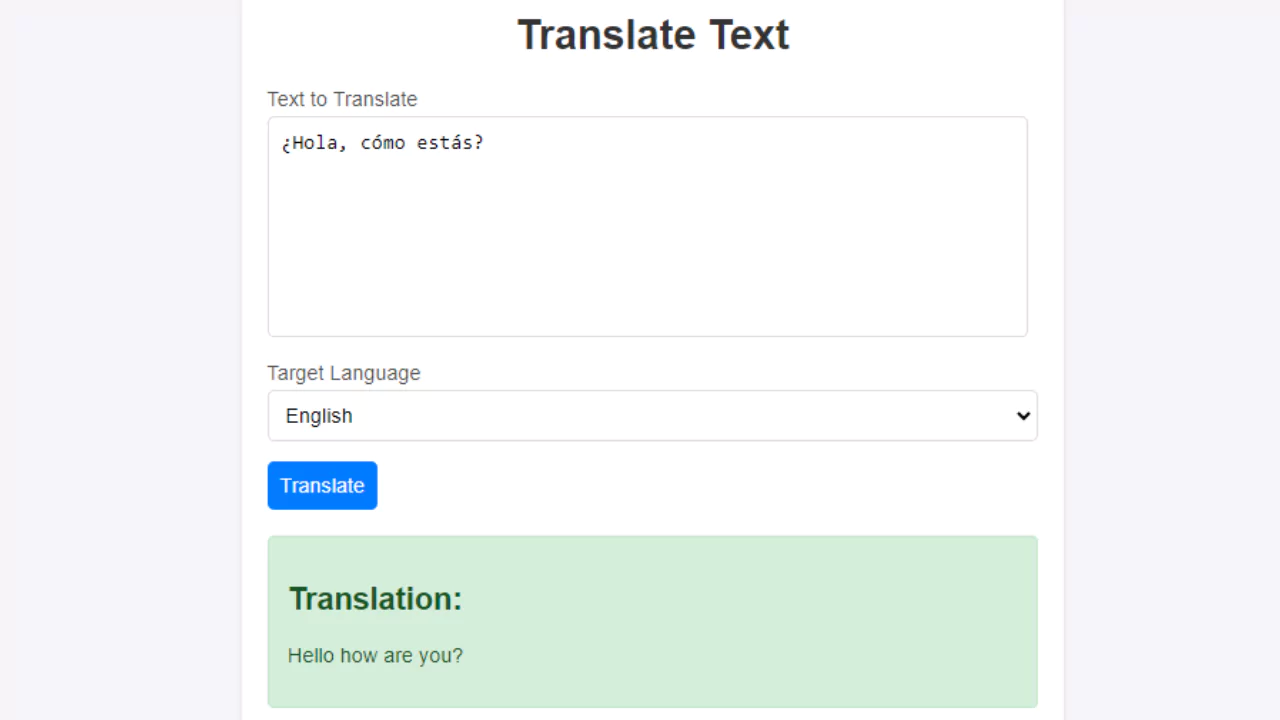
Create a translator web app in Django that allows users to translate text between different languages. This project will teach you how to integrate external APIs into your Django projects and handle multilingual content.
12. Authentication System
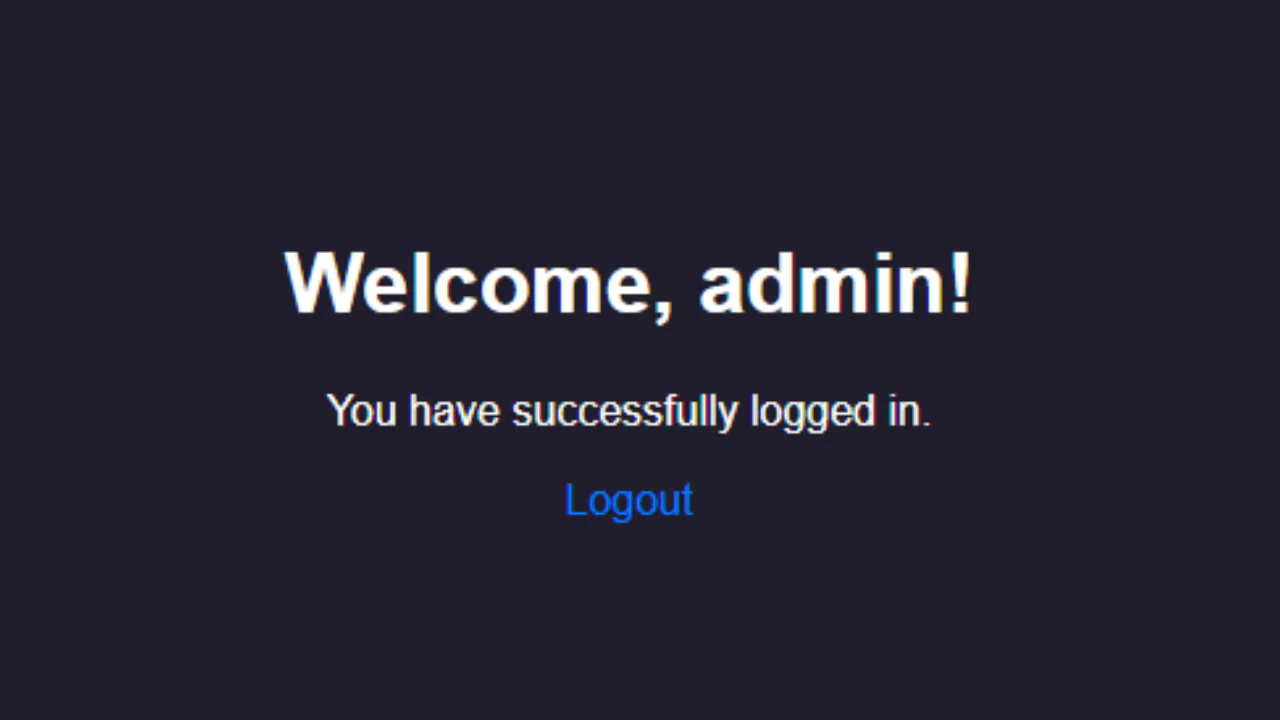
Develop a robust authentication system using Django, complete with user registration, login, and password management features. This project will provide a solid foundation in user authentication and security best practices in Django.
13. Text Utilities
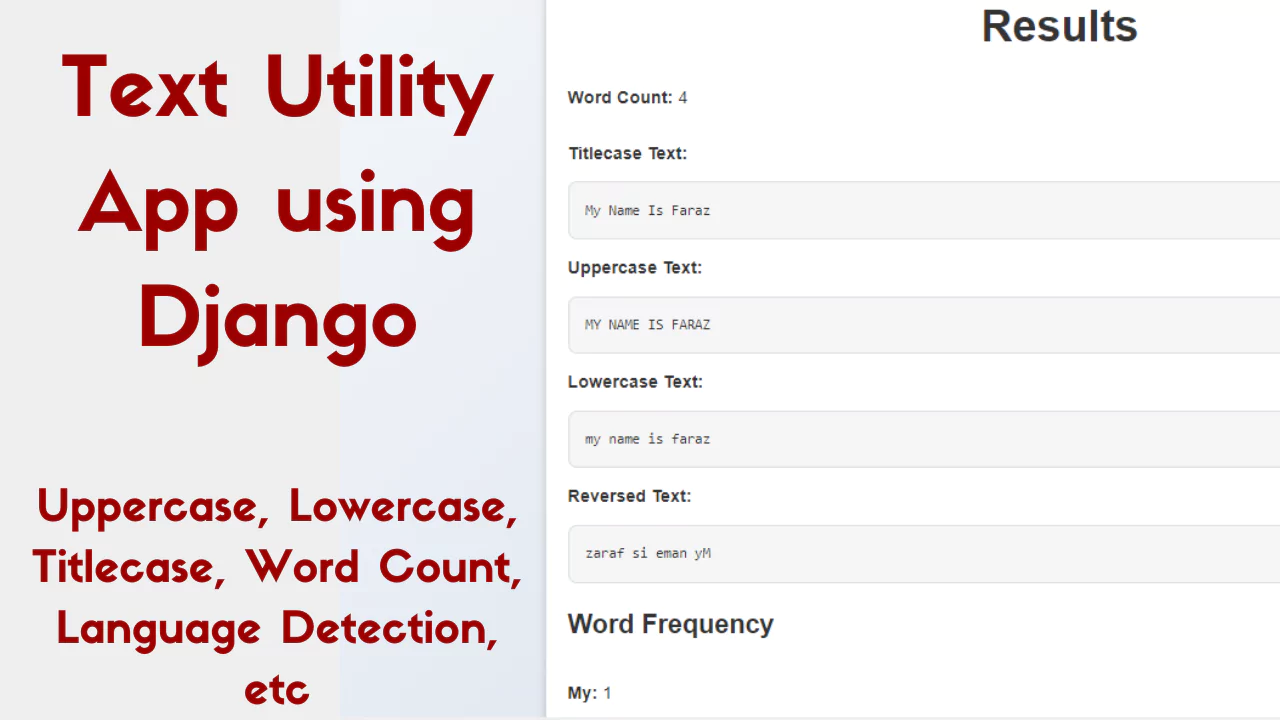
In this project, you'll create a set of text utilities in Django that allow users to convert text to uppercase, lowercase, and count words. This is a great way to learn about text manipulation and form handling in Django.
14. Shopping Cart
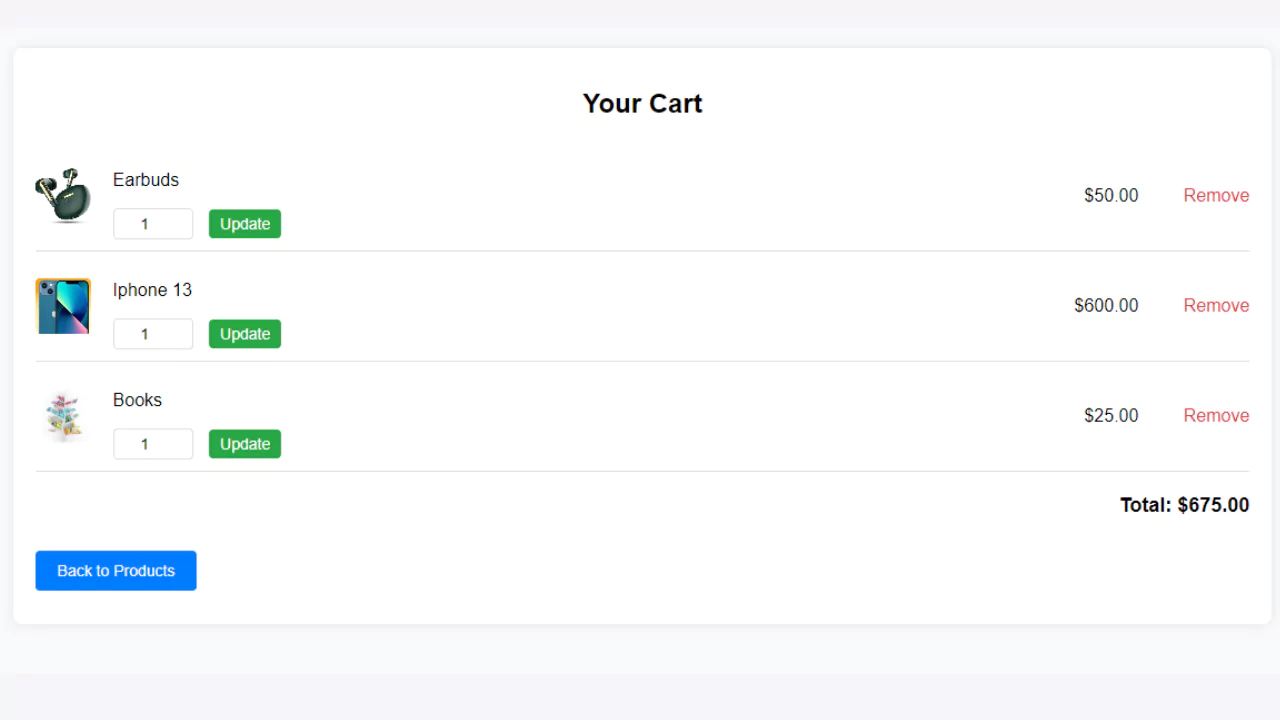
Build a shopping cart system for an e-commerce site, allowing users to add, remove, and manage items before checkout.
15. Weather App
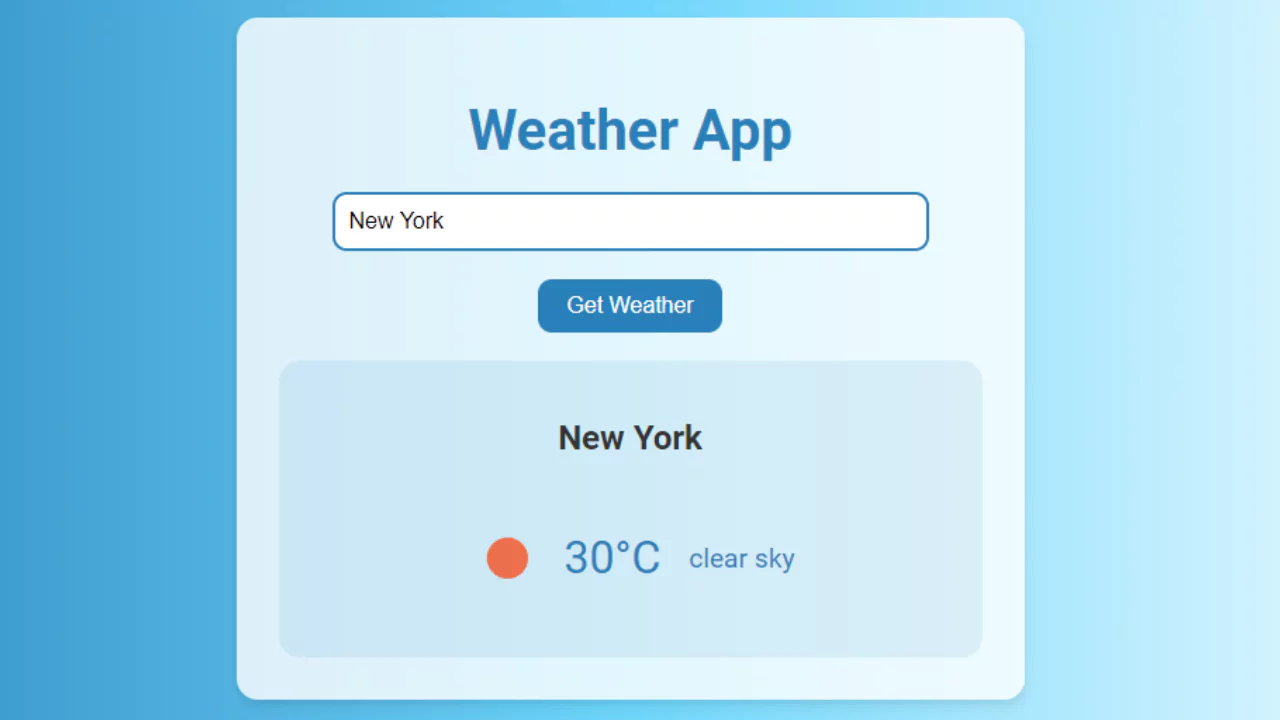
Create a weather app using Django that fetches and displays current weather data based on user input. This project is perfect for learning how to work with external APIs and present real-time data to users.
16. CRUD Application
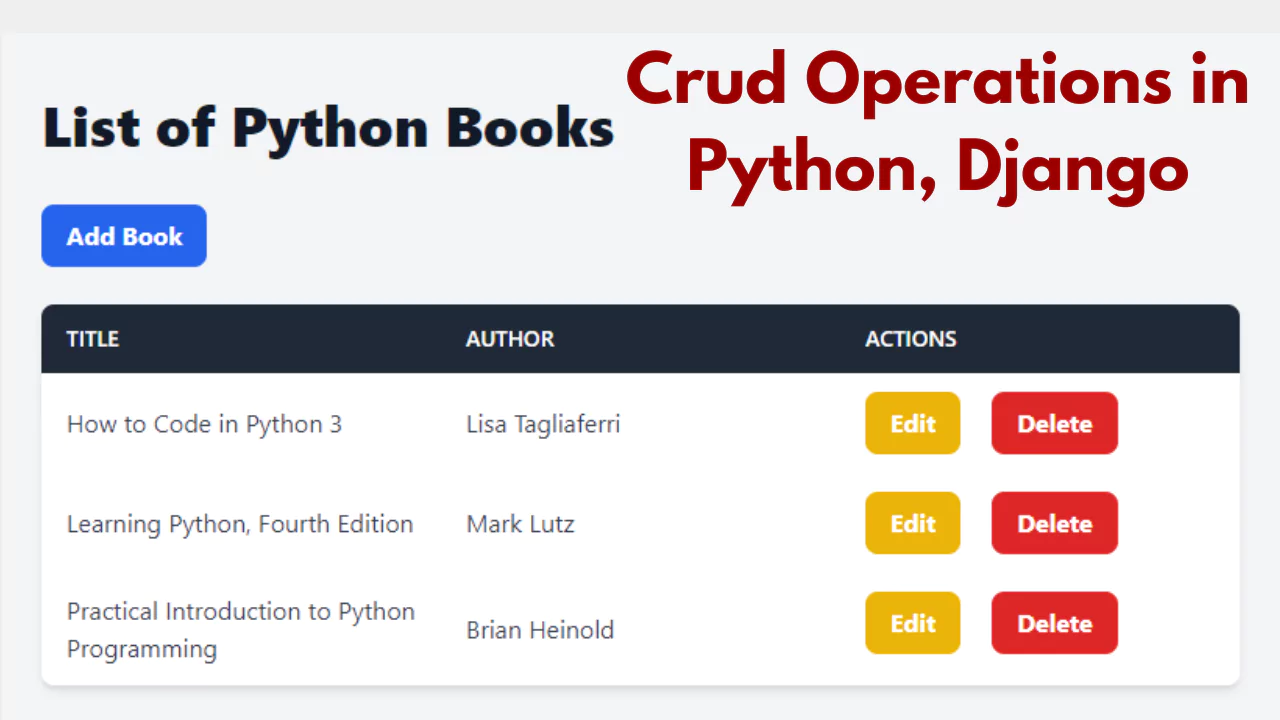
Create a full CRUD application using Django that allows users to manage a list of items. This project is essential for understanding the core concepts of Django, such as models, views, and templates.
17. TODO App
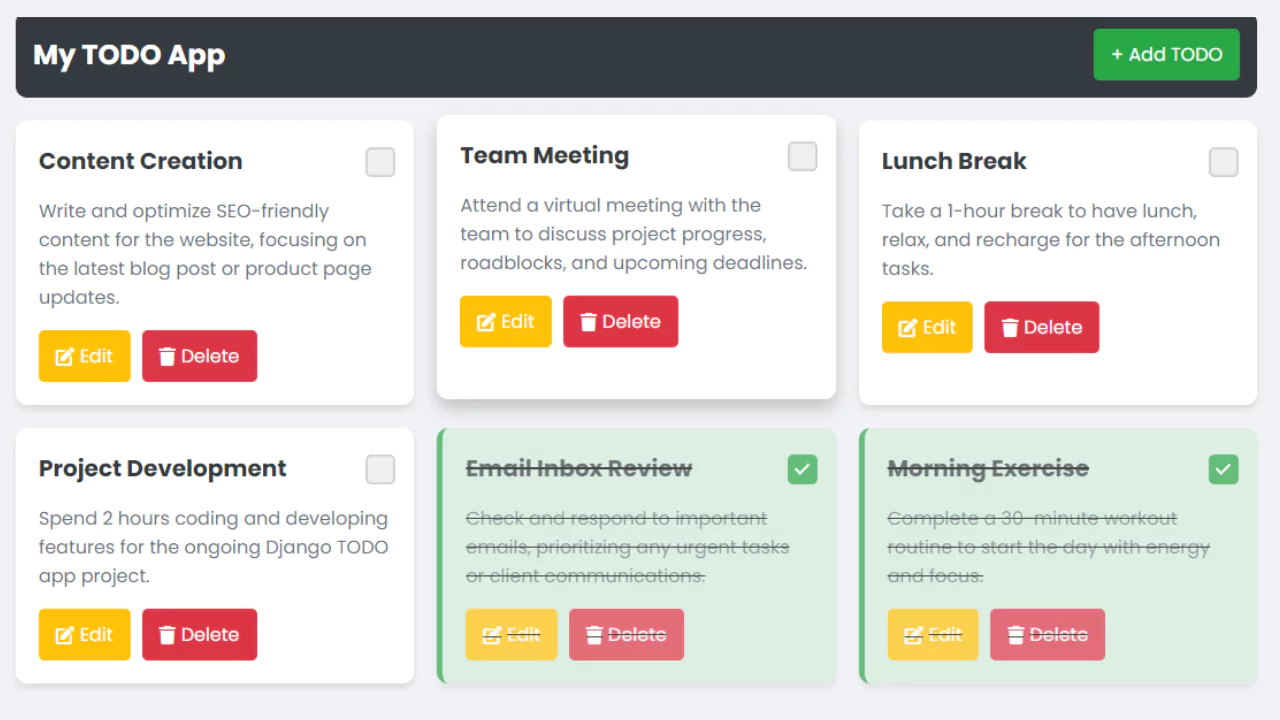
Develop a TODO application in Django where users can add, edit, and delete tasks. This project will help you understand CRUD (Create, Read, Update, Delete) operations and how to manage user data in Django.
18. Currency Converter
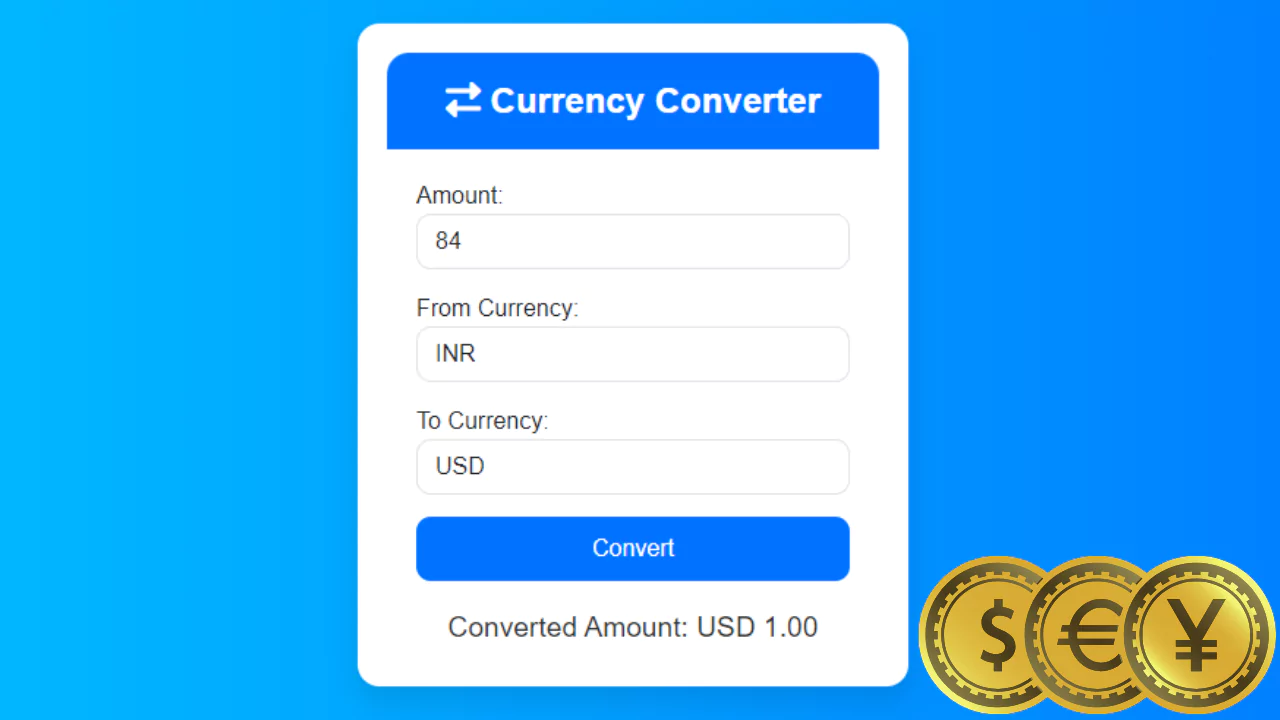
Build a currency converter using Django that converts amounts between different currencies based on the latest exchange rates. This project will introduce you to working with external APIs and managing financial data in Django.
19. Notes App
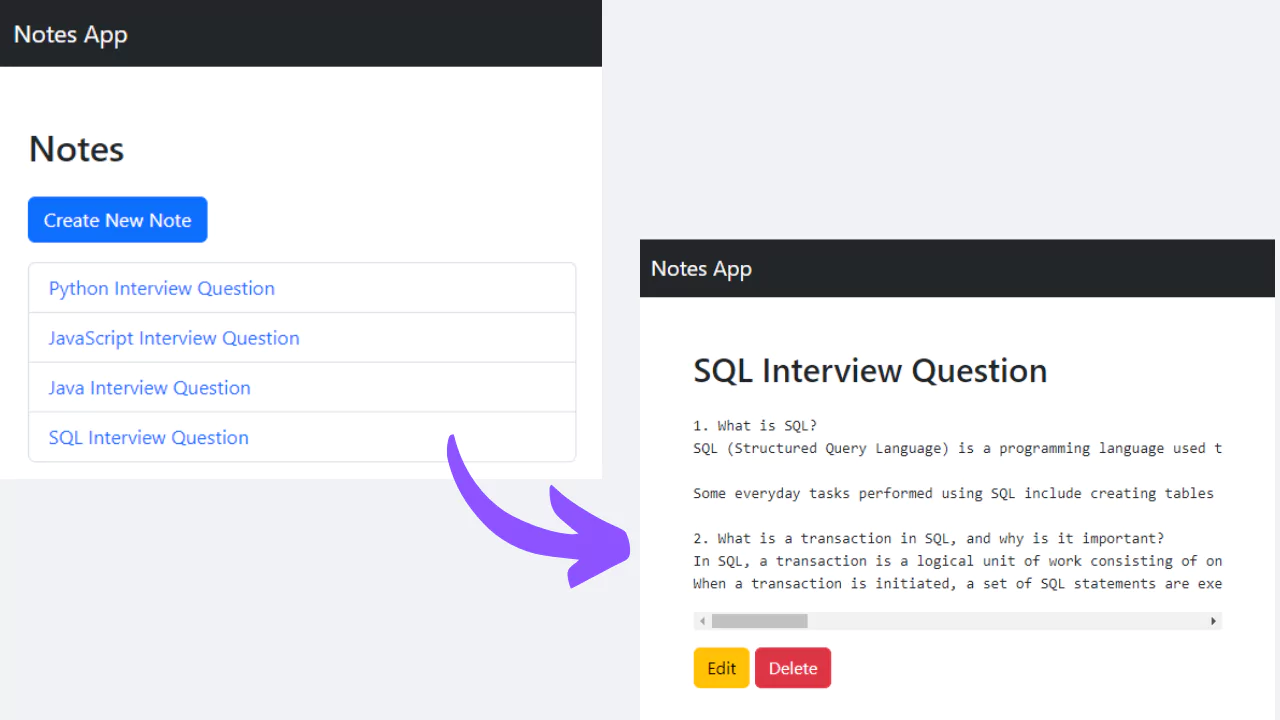
Develop a notes app in Django where users can create, edit, and organize their notes. This project is great for learning about user-generated content, data storage, and user interface design in Django.
20. Email Signature Generator
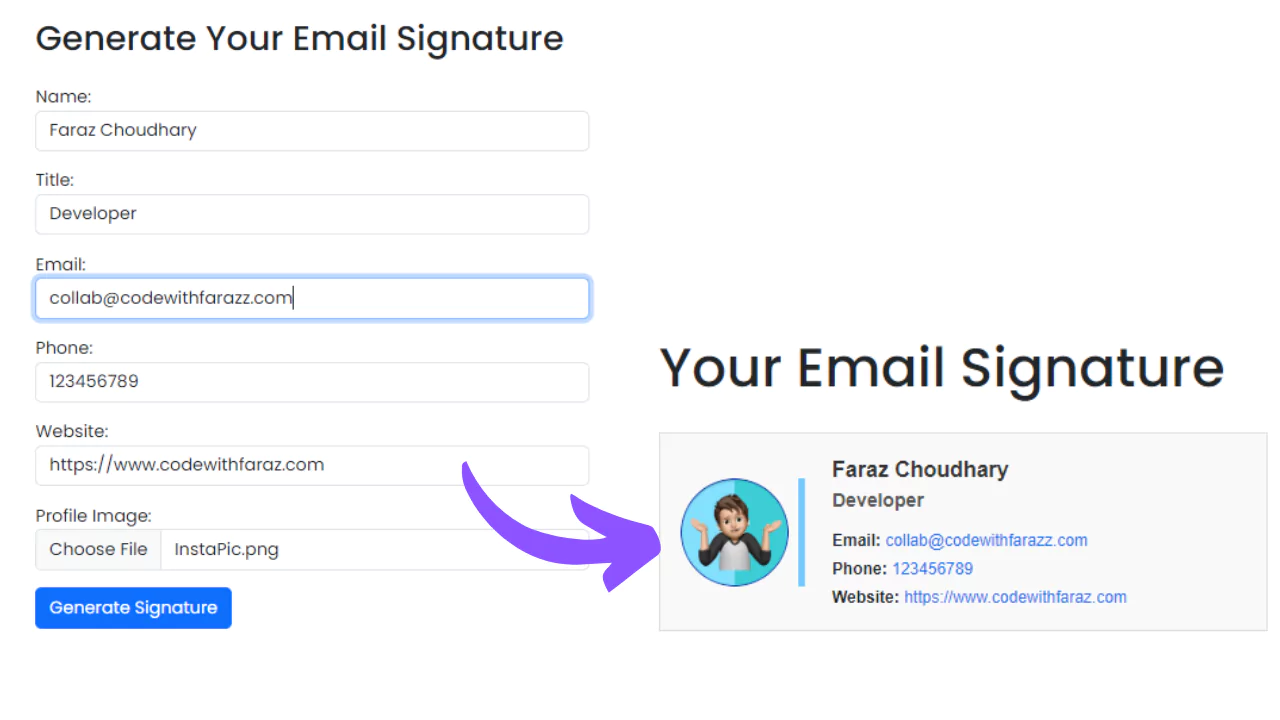
Create an email signature generator using Django that allows users to create custom signatures with text, images, and links. This project will help you understand how to handle and format user input in a visually appealing way.
21. Event Management System
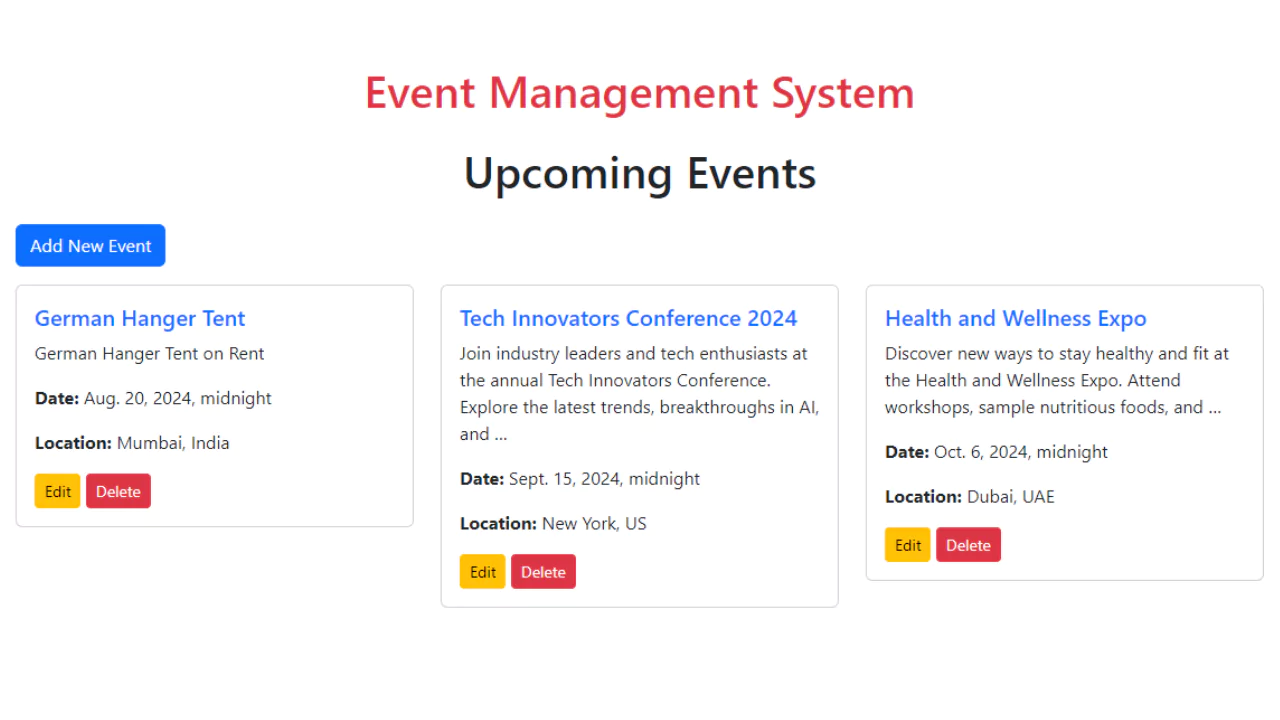
Build an event management system in Django where users can schedule events, manage attendees, and send invitations. This project will teach you about complex data relationships, user interaction, and real-time notifications in Django.
How to Run Your Server
Once your environment is set up and your project is created, you can run the Django development server by following these steps:
- Navigate to Your Project Directory:
- Apply Migrations:
- Django uses a database to store data. Run the following command to apply the initial migrations:
- Create a Superuser (Optional):
- If you want to access the Django admin panel, create a superuser by running:
- Follow the prompts to set up a username and password.
- Run the Server:
- To start the Django development server, use the command:
- By default, the server will run at http://127.0.0.1:8000/. Open this URL in your web browser to view your project.
cd myproject
python manage.py migrate
python manage.py createsuperuser
python manage.py runserver
Conclusion
Working on Django projects is an excellent way to build your skills and gain hands-on experience. These 20+ projects cover a wide range of applications, from simple to complex, and provide valuable learning opportunities. Whether you're looking to start your first Django project or enhance your existing knowledge, these projects and their source code will be a great resource. Dive in and start building today!
That’s a wrap!
Thank you for taking the time to read this article! I hope you found it informative and enjoyable. If you did, please consider sharing it with your friends and followers. Your support helps me continue creating content like this.
Stay updated with our latest content by signing up for our email newsletter! Be the first to know about new articles and exciting updates directly in your inbox. Don't miss out—subscribe today!
If you'd like to support my work directly, you can buy me a coffee . Your generosity is greatly appreciated and helps me keep bringing you high-quality articles.
Thanks!
Faraz 😊